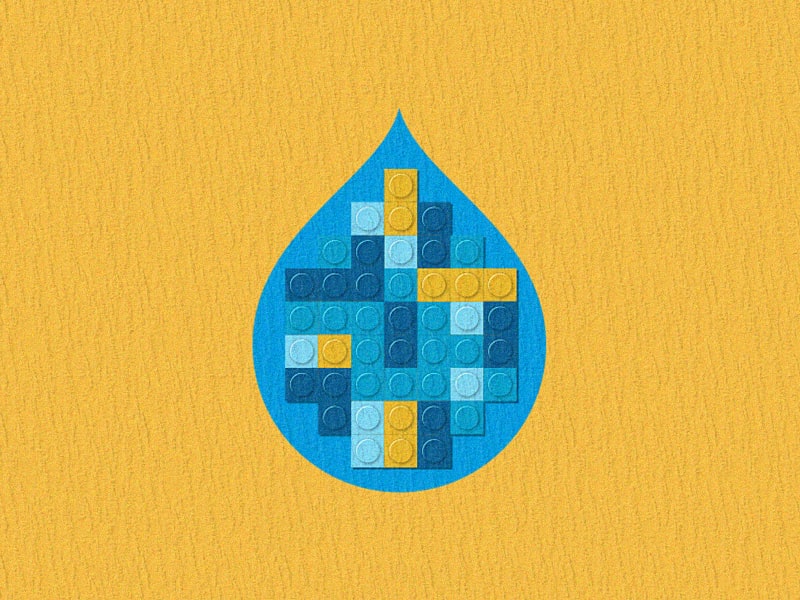
Blocks are an integral part of any Drupal website. They are chunks of content that can be placed in various regions and can be easily moved around the web page. Blocks can contain simple text, forms, or some complex logic. The Block module is a core module since Drupal 8 and simply needs to be enabled to get it to work. Sometimes, installing other core or contributed modules can automatically enable them too.
A block is a piece of content that can be positioned anywhere on your Drupal site. It can include simple text, forms, or complex logic. While Drupal offers a range of useful blocks for various scenarios, there are times when creating custom blocks is necessary.
What is a Block
The custom block is now visible in the region where it has been placed.
The annotation contains:
- Through the Drupal interface.
- Programmatically in code.
How to Create a Custom Block in Drupal
Create a module
<?php
namespace Drupalcustom_blockPluginBlock;
use DrupalCoreBlockBlockBase;
/**
* Provides a Custom block.
*
* @Block(
* id = "custom_block",
* admin_label = @Translation("Custom Block"),
* )
*/
class CustomBlock extends BlockBase { }
name: Custom Block
description: Defines a custom module.
type: module
core_version_requirement: ^9 || ^10
package: custom
dependencies:
- drupal:block
<?php
Create a Block Class
Custom blocks can be created in two ways:
namespace Drupalcustom_blockPluginBlock;
Here, the block is displayed on the node with ID 23 for every user. After adjusting the configuration as needed, click on “Save block” and return to the site.
- id: This is a unique, machine-readable ID for the custom block.
- admin_label: This is a human-readable name for the block, displayed in the admin interface.
Blocks are a core component of Drupal. While creating and managing custom blocks in Drupal 8 and Drupal 10 follow a similar process, Drupal 10 offers a more streamlined, optimized, and modern development experience with enhanced performance, security, and flexibility. Hope you found this tutorial helpful and that it gets you started with creating custom blocks in Drupal.
/**
* {@inheritdoc}
*/
public function access(AccountInterface $account, $return_as_object = false) {
// Check if the user has the 'access content' permission.
if ($account->hasPermission('access content')) {
return AccessResult::allowed();
}
return AccessResult::forbidden();
}
- blockForm() : This method is used to define a configuration form for a custom block, allowing admins to configure settings for the block while placing it in the block layout.
Inside the newly created custom_block folder, create a file named <module_name>.info.yml. Since the module name is custom_block, the file should be named custom_block.info.yml. Add the following contents to this file:The CustomBlock.php file should include annotation metadata to help identify the custom block. Annotations in Drupal are used for defining and identifying plugins like blocks, views, and more. For more information on annotations, you can refer to the “Annotations-based plugins” page on Drupal.org.
To create a custom block, you must first create a .info.yml file in the modules/custom directory. If the custom directory does not exist, you will need to create it. Start by creating a directory named custom inside the modules directory. Next, create another directory named custom_block within modules/custom. The name of this directory will correspond to the name of the module you are creating.
Enable the Block to display content
- After saving the file, go to Admin > Structure > Block Layout
- Click on Place block under the region where you want the block to appear. In this case, the block is placed in the Content region.
- After clicking on “Place block,” search for the custom block you created.
- Click on “Place block” for the block you want to display. This will open the configuration window, where you can adjust the settings as needed.
use DrupalCoreBlockBlockBase;
To define the block’s logic, we need to create a Drupal block class. The PHP class for the block should be placed in the modules/custom/custom_block/src/Plugin/Block directory. Create this folder structure and add a class named CustomBlock.php within the Block directory.
Methods used to create custom blocks
- build() : This method returns a renderable array. In this example, it returns simple markup, but it can also be used to return more complex content, such as forms and views.
- blockAccess() : This method defines custom access logic for users.
If you’re looking for Drupal development services to create powerful digital solutions, Specbee is here to help! Talk to us today.
Final thoughts
/**
* Provides a Custom block.
*
* @Block(
* id = "custom_block",
* admin_label = @Translation("Custom Block"),
* )
*/
class CustomBlock extends BlockBase {
/**
* {@inheritdoc}
*/
public function build() {
return [
'#markup' => $this->t('This is a custom block.'),
];
}
}
Although these blocks can be modified to suit the layout, complete flexibility and customization can only be achieved with custom blocks. You can create a custom block programmatically or via the Drupal Interface. In this article, we will take you through the process of creating a block programmatically and enabling it through the admin interface.